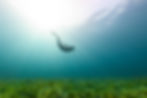
In today's digital age, mobile apps play a significant role in our lives. However, many app developers often overlook the importance of accessibility. Building mobile apps with accessibility in mind ensures that everyone, including individuals with disabilities, can access and enjoy your app without barriers. Flutter, a popular cross-platform framework, offers several features and tools to create accessible mobile apps.
In this blog, we will explore some essential accessibility guidelines for developing mobile apps with Flutter and provide example code to demonstrate each guideline.
1. Provide Meaningful Semantics
To make your app more accessible, it's crucial to use proper semantics for widgets and elements. Semantics help screen readers understand the purpose and function of each UI component.
Example: Suppose you have a custom button in your app. Use the Semantics widget to provide meaningful semantics.
Semantics(
label: 'Submit Button',
child: ElevatedButton(
onPressed: () {
// Button click logic
},
child: Text('Submit'),
),
)
2. Use Descriptive Alt Text for Images
Images are a vital part of mobile apps, but they must be accessible to users who cannot see them. Providing descriptive alternative text (alt text) for images is essential for screen readers to convey the image's content.
Example: When using an image in your app, add an Image widget with the semanticLabel parameter:
Image(
image: AssetImage('assets/image.png'),
semanticLabel: 'A beautiful sunset at the beach',
)
3. Ensure Sufficient Contrast
Maintaining proper contrast between text and background is crucial for users with visual impairments. Flutter provides a ThemeData class that allows you to define consistent colors throughout your app and adhere to accessibility standards.
Example: Define a custom theme with sufficient contrast:
ThemeData(
brightness: Brightness.light,
primaryColor: Colors.blue,
accentColor: Colors.orange,
textTheme: TextTheme(
bodyText1: TextStyle(color: Colors.black87),
bodyText2: TextStyle(color: Colors.black54),
),
)
4. Enable built-in Screen Reader Support in Flutter
Flutter has built-in support for screen readers like TalkBack (Android) and VoiceOver (iOS). To enable screen reader support, ensure that your UI components are accessible and convey the relevant information to the users.
Example: For adding accessibility support to a text widget:
Text(
'Hello, World!',
semanticsLabel: 'Greeting',
)
5. Manage Focus and Navigation
Proper focus management is crucial for users who rely on keyboard navigation or screen readers. Ensure that focus is visible and logical when navigating through your app's elements.
Example: Implement a FocusNode and Focus widget to manage focus:
class FocusDemo extends StatefulWidget {
@override
_FocusDemoState createState() => _FocusDemoState();
}
class _FocusDemoState extends State<FocusDemo> {
final FocusNode _focusNode = FocusNode();
@override
Widget build(BuildContext context) {
return Focus(
focusNode: _focusNode,
child: ElevatedButton(
onPressed: () {
// Button click logic
},
child: Text('Click Me'),
),
);
}
}
6. Handle Dynamic Text Sizes
Some users may rely on larger text sizes for better readability. Flutter supports dynamic text sizes that adapt to the user's accessibility settings.
Example: Use the MediaQuery to access the user's text scale factor:
dartCopy code
Text(
'Dynamic Text',
style: TextStyle(fontSize: MediaQuery.of(context).textScaleFactor * 20),
)
Conclusion
Building accessible mobile apps with Flutter is not only a legal and ethical obligation but also a step towards creating a more inclusive digital environment. By following the guidelines mentioned in this blog, you can ensure that your app is accessible to a broader audience, including individuals with disabilities.
Remember that accessibility is an ongoing process, and continuous user feedback and testing are essential to refine your app's accessibility. Let's strive to make technology more inclusive and accessible for everyone!