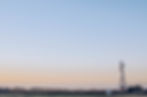
In Swift, structs are an essential feature of the language that allows developers to create custom data types to encapsulate related pieces of data and functionality. Unlike classes, structs are value types, meaning they are copied when passed around, which has numerous advantages.
In this blog, we'll explore the benefits of using structs in Swift and provide insights into how to use them effectively in your code.
Advantages of Using Structs
1. Value Semantics
One of the most significant advantages of using structs is their value semantics. When you create an instance of a struct and assign it to another variable or pass it as a parameter to a function, a complete copy of the struct is made. This behavior eliminates issues related to shared mutable state, making code more predictable and less prone to bugs.
struct Point {
var x: Int
var y: Int
}
var point1 = Point(x: 10, y: 20)
var point2 = point1 // Creates a copy of the struct
point2.x = 100 // Only modifies point2, leaving point1 unchanged
2. Performance and Memory Efficiency
Since structs are copied by value, they are stored directly where they are used, usually on the stack. This allocation strategy results in better memory management and performance compared to reference types (classes) that use heap storage. Structs are particularly useful for small, lightweight data types, which are prevalent in many applications.
3. Thread Safety
Due to their immutability and value semantics, structs are inherently thread-safe. Since they cannot be mutated once created, they eliminate the need for synchronization mechanisms like locks or serial dispatch queues in concurrent programming scenarios.
4. Swift Standard Library Foundation
Many essential Swift types, such as Int, Double, Bool, String, Array, and Dictionary, are implemented as structs in the Swift Standard Library. Leveraging structs enables you to build on top of these foundational types effectively.
5. Copy-on-Write Optimization
Swift's copy-on-write optimization further enhances the performance of structs. When a copy of a struct is made, the actual data is not duplicated immediately. Instead, both copies share the same data. The data is only duplicated when one of the copies is modified, ensuring efficient memory management.
Effective Usage of Structs
1. Model Data
Structs are ideal for modeling data, especially when dealing with simple objects with no need for inheritance or identity. For example, consider using structs to represent geometric shapes, user profiles, or configuration settings.
struct Circle {
var radius: Double
var center: Point
}
struct UserProfile {
var username: String
var email: String
var age: Int
}
2. Immutability
Consider making structs immutable whenever possible. Immutable structs prevent accidental modifications, leading to more robust and predictable code.
struct ImmutablePoint {
let x: Int
let y: Int
}
3. Small-sized Data Structures
As mentioned earlier, structs are great for small-sized data structures. For larger and more complex data structures, classes might be a more appropriate choice.
4. Use Extensions for Additional Functionality
To keep the primary purpose of a struct focused and maintain separation of concerns, use extensions to add extra functionality.
struct Point {
var x: Int
var y: Int
}
extension Point {
func distance(to otherPoint: Point) -> Double {
let xDist = Double(x - otherPoint.x)
let yDist = Double(y - otherPoint.y)
return (xDist * xDist + yDist * yDist).squareRoot()
}
}
5. Use Mutating Methods Sparingly
If you need to modify a struct, you must declare the method as mutating. However, try to limit the number of mutating methods and prefer immutability whenever possible.
Conclusion
Swift structs offer numerous advantages, including value semantics, performance, thread safety, and easy integration with the Swift Standard Library. By using structs effectively, you can write more robust, predictable, and efficient code. Remember to choose structs when modeling small-sized data and prefer immutability for improved code safety. Swift's powerful language features, combined with the advantages of structs, make it a great choice for developing applications across various domains.
Remember to practice and experiment with structs in your code to gain a deeper understanding of their advantages and to leverage their capabilities effectively.
Happy coding!