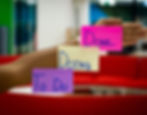
CoroutineWorker is a Kotlin-specific class in Android WorkManager that allows you to run asynchronous tasks in the background. It is a wrapper around the Worker class, and it provides a number of benefits, including:
Support for Kotlin Coroutines: CoroutineWorker uses Kotlin Coroutines to run asynchronous tasks, which makes your code more concise and expressive.
Automatic handling of stoppages and cancellation: CoroutineWorker automatically handles stoppages and cancellation, so you don't have to worry about manually managing these states.
Better performance: CoroutineWorker can often perform better than the Worker class because it uses Kotlin Coroutines to manage its state.
To take advantage of the latest Android features: CoroutineWorker is a newer class than the Worker class, and it supports some of the latest Android features, such as Kotlin Flow.
Implementing CoroutineWorker
To use CoroutineWorker, you first need to create a subclass of the CoroutineWorker class. In your subclass, you need to implement the doWork() method. This method is where you will write your asynchronous code.
Here is a simple example of a CoroutineWorker subclass:
class MyCoroutineWorker : CoroutineWorker() {
override suspend fun doWork(): Result {
// Perform your asynchronous task here.
return Result.success()
}
}
Once you have created your CoroutineWorker subclass, you can schedule it to run using the WorkManager class.
Here is an example of how to schedule a CoroutineWorker to run:
val work = OneTimeWorkRequestBuilder<MyCoroutineWorker>()
.build()
WorkManager.getInstance(context).enqueue(work)
Scheduling Tasks with CoroutineWorker
You can do this by using the PeriodicWorkRequestBuilder class. This class allows you to create a WorkRequest that will be executed periodically at a specified interval.
Here is an example of how to schedule a task to run periodically with CoroutineWorker:
val work = PeriodicWorkRequestBuilder<MyCoroutineWorker>(
repeatInterval = Duration.ofHours(1)
).build()
WorkManager.getInstance(context).enqueue(work)
This code will schedule a CoroutineWorker to run every hour. The CoroutineWorker will be executed in the background, and it will be able to access all of the same resources as a regular CoroutineWorker.
You can also schedule tasks with CoroutineWorker to run only once. To do this, you can use the OneTimeWorkRequestBuilder class. This class allows you to create a WorkRequest that will be executed once and then discarded.
When to use CoroutineWorker
You should use CoroutineWorker whenever you need to run an asynchronous task in the background. This includes tasks such as:
Downloading data from the internet
Uploading data to the cloud
Performing database queries
Processing images
Sending notifications
CoroutineWorker is a good choice for these types of tasks because it is easy to use, efficient, and reliable.
Limitations of CoroutineWorker
Here are some cases where you should not use CoroutineWorker:
If you need to run a task that requires a lot of CPU or memory resources. CoroutineWorker is designed for running lightweight background tasks. If you need to run a task that requires a lot of resources, you should use a different approach, such as starting a service or using a foreground process.
If you need to run a task that needs to be able to interact with the UI. CoroutineWorker runs in the background, so it cannot interact with the UI directly. If you need to run a task that needs to be able to interact with the UI, you should use a different approach, such as using a worker thread or posting a message to the main thread.
If you need to run a task that is critical to the user experience. CoroutineWorker does not guarantee that tasks will be executed in a timely manner. If you need to run a task that is critical to the user experience, you should use a different approach, such as using a service or using a foreground process.
Overall, CoroutineWorker is a good choice for running lightweight background tasks in Android apps. However, it is important to be aware of its limitations and to use it in the appropriate scenarios.