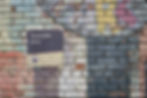
In today's diverse and inclusive world, it's essential to design and develop apps that are accessible to individuals with disabilities.
In this blog, we'll explore how to create iOS apps that prioritize accessibility, ensuring that every user can enjoy and navigate through your app seamlessly. We'll cover important aspects such as accessibility APIs, VoiceOver support, dynamic type, accessible layout, and assistive technologies using Swift and SwiftUI code examples.
1. Understanding Accessibility in iOS Apps
Accessibility is about making your app usable and navigable by people with various disabilities, such as visual impairments, hearing impairments, motor skill limitations, and more. By following accessibility best practices, you can enhance your app's user experience and make it inclusive to a wider audience.
2. Setting Up Accessibility in Your Project
In Xcode, when you create a new project, you'll find an option to enable accessibility. Ensure that this option is selected from the beginning to set up the project with accessibility support.
3. Accessibility APIs
iOS provides a range of Accessibility APIs that developers can use to make their apps accessible. Some of the most commonly used APIs include:
UIAccessibility: This protocol helps to identify and describe the elements of your UI to assistive technologies. Conform to this protocol in custom views to provide relevant accessibility information.
UIAccessibilityElement: Implement this class to create custom accessibility elements within your views. It allows you to provide custom accessibility traits, labels, and hints.
4. VoiceOver Support
VoiceOver is a built-in screen reader on iOS devices that reads the content of the screen aloud, making it accessible to users with visual impairments. Ensure your app works seamlessly with VoiceOver by:
Providing meaningful accessibility labels: Use the accessibilityLabel property on UI elements to give descriptive labels to buttons, images, and other interactive elements.
Adding accessibility hints: Use the accessibilityHint property to provide additional context or instructions for VoiceOver users.
Example:
import SwiftUI
struct AccessibleButton: View {
var body: some View {
Button(action: {
// Your button action here
}) {
Text("Tap me")
.accessibilityLabel("A button that does something")
.accessibilityHint("Double-tap to activate")
}
}
}
5. Dynamic Type
iOS supports Dynamic Type, which allows users to adjust the system font size according to their preferences. To ensure your app is compatible with Dynamic Type, use system fonts and prefer relative font weights. Avoid hardcoding font sizes.
Example:
swiftCopy code
import SwiftUI
struct AccessibleText: View {
var body: some View {
Text("Hello, World!")
.font(.title)
.fontWeight(.bold)
.multilineTextAlignment(.center)
.lineLimit(0)
.padding()
.minimumScaleFactor(0.5) // Allows text to scale down for smaller fonts
.allowsTightening(true) // Allows letters to tighten when necessary
}
}
6. Accessible Layout
An accessible layout is crucial for users with motor skill impairments or those who use alternative input devices. Ensure that your app's user interface is designed with sufficient touch target size, making it easier for users to interact with buttons and controls.
Example:
import SwiftUI
struct AccessibleList: View {
var body: some View {
List {
ForEach(0..<10) { index in
Text("Item \(index)")
.padding()
.contentShape(Rectangle()) // Increase the tappable area for VoiceOver users
}
}
}
}
7. Testing with Assistive Technologies
Test your app's accessibility using assistive technologies such as VoiceOver, Switch Control, and Zoom. Put yourself in the shoes of users with disabilities to identify and fix potential accessibility issues.
Conclusion
In this blog, we've explored the key elements of creating accessible iOS apps using Swift and SwiftUI. By embracing accessibility APIs, supporting VoiceOver, implementing Dynamic Type, designing an accessible layout, and testing with assistive technologies, you can make your app inclusive and enrich the user experience for everyone. Prioritizing accessibility is not only a legal and ethical responsibility but also a great way to expand your app's user base and contribute to a more inclusive world.