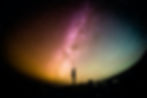
Dio is a popular HTTP client library for Dart and Flutter. It provides a comprehensive and high-performance API for making HTTP requests, with support for multiple core features.
Why use Dio in Flutter?
Dio offers a number of advantages over the built-in http package in Flutter, including:
More features: Dio provides a wider range of features than the http package, such as global configuration, interceptors, and request cancellation.
Better performance: Dio is generally considered to be more performant than the http package, especially for complex requests.
Easier to use: Dio provides an intuitive and easy-to-use API, making it a good choice for both beginners and experienced developers.
How to integrate Dio with a Flutter project
To integrate Dio with a Flutter project, you can follow these steps:
Add the Dio dependency to your pubspec.yaml file:
dependencies:
dio: ^5.3.3
Run flutter pub get to install the Dio package.
Create a new Dio instance:
import 'package:dio/dio.dart';
class MyApiClient {
final dio = Dio();
}
Make HTTP requests using the Dio instance:
Future<Response> get(String url) async {
return await dio.get(url);
}
Future<Response> post(String url, dynamic data) async {
return await dio.post(url, data: data);
}
Handle errors:
try {
Response response = await dio.get(url);
// Handle the response
} catch (e) {
// Handle the error
}
Features of the Dio plugin
Dio provides a number of features that make it a powerful and versatile HTTP client for Flutter, including:
Global configuration:Dio allows you to set global configurations that apply to all requests made by the client. This includes options like setting default headers, base URLs, and more.
Interceptors: Dio supports interceptors, which allow you to intercept and modify requests and responses. This can be used to implement features such as authentication, logging, and caching.
Request cancellation: Dio allows you to cancel requests in progress. This can be useful if you need to stop a request that is no longer needed.
File downloading: Dio provides a built-in file downloader that can be used to download files from the server.
Timeout: Dio allows you to set a timeout for requests. This can be useful to prevent requests from hanging indefinitely.
Disadvantages of using Dio over http in Flutter
Dio has a few potential disadvantages over the built-in http package in Flutter, including:
Larger package size: The Dio package is larger than the http package, which can increase the size of your Flutter app.
Steeper learning curve: Dio provides more features than the http package, which can make it more difficult to learn.
Community support: The http package is more widely used than Dio, so there is a larger community of developers who can provide support.
Overall, Dio is a powerful and versatile HTTP client for Flutter that offers a number of advantages over the built-in http package.