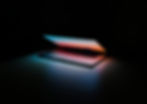
Security is an essential aspect of iOS app development. Swift, being a relatively new programming language, provides developers with a modern, secure, and robust platform to build applications. However, even with Swift, there are still security considerations to keep in mind when developing iOS apps.
In this blog post, we will discuss the security considerations for iOS app development using Swift and provide code samples to demonstrate how to implement them.
1. Secure Communication
Communication is a crucial aspect of any mobile application, and it is vital to ensure that communication channels are secure. When developing an iOS app, you should use secure protocols such as HTTPS to communicate with remote servers. HTTPS provides encryption for data in transit, protecting it from interception or tampering.
To use HTTPS in your iOS app, you can use the URLSession class in Swift. Here's an example of how to create a URLSession with a secure HTTPS configuration:
let configuration = URLSessionConfiguration.default
configuration.httpAdditionalHeaders = ["Accept": "application/json"]
configuration.requestCachePolicy = .reloadIgnoringLocalCacheData
configuration.urlCache = nil
let session = URLSession(configuration: configuration, delegate: nil, delegateQueue: OperationQueue.main)
In this example, we set the httpAdditionalHeaders to accept JSON data, and we configure the URL cache policy to ignore any local cache data. We also set the URL cache to nil to ensure that no cached data is stored on the device.
2. Authentication and Authorization
Authentication and authorization are essential security considerations when developing an iOS app. You should always authenticate users before allowing them access to your app's sensitive data or features. There are various authentication methods you can use in your iOS app, such as passwords, biometric authentication, or OAuth2.
To implement password authentication in your iOS app, you can use Apple's built-in Keychain Services framework. The Keychain Services framework provides secure storage for sensitive information, such as passwords, and ensures that the data is encrypted and protected.
Here's an example of how to use Keychain Services to store and retrieve a user's password:
let keychain = Keychain(service: "com.yourapp.yourappname")
// Store the password
do {
try keychain.set("password", forKey: "username")
} catch let error {
print("Unable to store password: \(error)")
}
// Retrieve the password
do {
let password = try keychain.get("username")
print("Password: \(password)")
} catch let error {
print("Unable to retrieve password: \(error)")
}
In this example, we create a Keychain instance for our app and store a password for a username. We then retrieve the password using the same username.
3. Data Protection
Data protection is another critical aspect of iOS app development. You should always ensure that sensitive data is encrypted and protected, both in transit and at rest. You can use Swift's built-in encryption classes to encrypt data, such as the CommonCrypto library.
Here's an example of how to encrypt and decrypt data using CommonCrypto in Swift:
// Encryption
let data = "My sensitive data".data(using: .utf8)!
let key = "My encryption key".data(using: .utf8)!
let encryptedData = try! AES256.encrypt(data: data, key: key)
// Decryption
let decryptedData = try! AES256.decrypt(data: encryptedData, key: key)
let decryptedString = String(data: decryptedData, encoding: .utf8)!
In this example, we use the AES256 encryption algorithm to encrypt some sensitive data using a key. We then decrypt the data using the same key to retrieve the original data.
4. Code Obfuscation
Code obfuscation is the process of making the source code difficult to understand or reverse-engineer. Obfuscation is particularly useful in preventing attackers from discovering vulnerabilities in your app's code or using your app's functionality without permission.
To obfuscate your Swift code, you can use tools such as Obfuscator-iOS, SwiftShield, or iXGuard. These tools can help protect your app from attackers who attempt to reverse-engineer your app's code.
5. Input Validation
Input validation is an essential security consideration in iOS app development. Input validation helps to ensure that users cannot enter invalid or malicious data into your app. If your app accepts user input, you should always validate the input to ensure that it meets your app's requirements.
Here's an example of how to validate user input in Swift using regular expressions:
func isValidEmail(email: String) -> Bool {
let emailRegex = "[A-Z0-9a-z._%+-]+@[A-Za-z0-9.-]+\\.[A-Za-z]{2,64}"
let emailPredicate = NSPredicate(format:"SELF MATCHES %@", emailRegex)
return emailPredicate.evaluate(with: email)
}
In this example, we use a regular expression to validate the format of an email address. We then use the NSPredicate class to evaluate whether the email address matches the regular expression.
Conclusion
In conclusion, security is an essential aspect of iOS app development using Swift. To ensure that your app is secure, you should implement secure communication, authentication and authorization, data protection, code obfuscation, and input validation. By following these security considerations and best practices, you can help protect your app and its users from various security threats.