Five ways to reduce your Android app size
- Don Peter
- Apr 27, 2023
- 4 min read
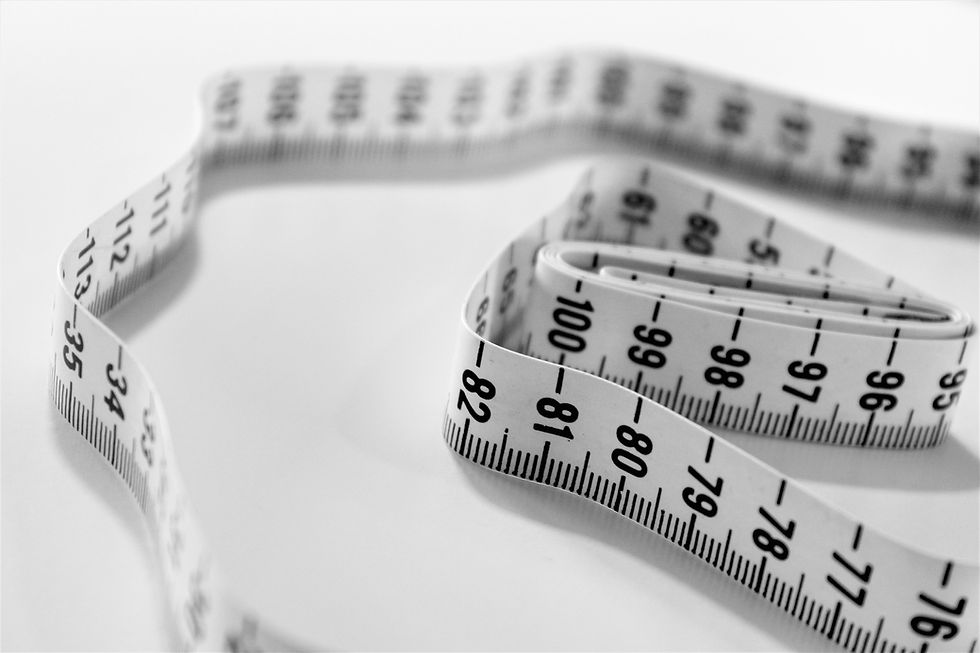
As an Android developer, one of the critical factors to consider when developing an app is its size. The smaller the size of your app, the better its chances of gaining more downloads and retaining users. A large app size can significantly impact user experience, particularly for those with limited storage on their devices.
In this post, we will discuss five ways to reduce Android app size without compromising functionality and performance.
1. Use Android App Bundle (AAB)
The Android App Bundle is a publishing format that helps reduce app size by delivering only the code and resources necessary for a particular device configuration. AAB is Google's recommended publishing format and is now required for all new apps on the Google Play Store.
Follow these steps to create an App Bundle:
1. Open your app-level build.gradle file.
2. Add the following code to the android block:
bundle {
language {
enableSplit = true
}
density {
enableSplit = true
}
abi {
enableSplit = true
}
}
3. Set the android.defaultConfig block to use the aab format:
android {
...
defaultConfig {
...
// Use the AAB format
bundle {
enabled = true
...
}
}
...
}
Finally, build and generate the Android App Bundle file by selecting "Build > Generate Signed Bundle/APK" in the Android Studio menu and selecting "Android App Bundle" as the build format.
2. Optimize images and graphics
Images and graphics can significantly increase the size of your app, particularly if they are not optimized. Consider using tools like TinyPNG or Compressor.io to compress your images and reduce their size without affecting their quality.
Using WebP images is an effective way to optimize images and graphics in your Android app. WebP is a modern image format developed by Google that provides superior compression compared to traditional image formats like JPEG and PNG. Using WebP images in your app can significantly reduce its size while maintaining high-quality images.
You can make use of inbuilt tool in Android studio to convert images to WebP format. Additionally, you can use vector images instead of bitmap images, as they are smaller and scale better across different device resolutions.
3. Minimize code and resources
Eliminate any unused code and resources from your app, as they can significantly increase its size. Use tools like ProGuard or R8 to remove unused code during the build process.
android {
buildTypes {
release {
minifyEnabled true
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
}
Additionally, use the 'shrinkResources' attribute in your build.gradle file to remove unused resources, such as icons and images, from your app.
android {
buildTypes {
release {
minifyEnabled true
shrinkResources true
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
}
4. Reduce the number of libraries
Each library you add to your app comes with its own set of resources, which can significantly increase your app size. Consider only using essential libraries and optimizing them to reduce their size. Here are some ways to help you reduce the number of libraries in your app:
Use only necessary libraries: Only use libraries that are essential to your app's functionality. Avoid using libraries that have overlapping functionality or libraries that you're not sure you need.
Evaluate the size of libraries and find lightweight alternatives: When considering using a library, evaluate its size and determine if it's worth the added weight to your app. Keep in mind that each library you add to your app adds to the total size of your APK. Whenever possible, use lightweight alternatives to larger libraries. For example, you could use a smaller library for JSON parsing instead of a larger library that includes other features you don't need.
5. Use dynamic features
Dynamic features are a new feature in the Android App Bundle that allows you to add features to your app dynamically, reducing the overall size of your app. For example, if your app has a feature that is only used by a small percentage of users, you can create a dynamic feature that is only downloaded when a user requests it, rather than including it in the initial app download.
Here's an example of how to implement dynamic features in your Android app:
Create a dynamic feature module: To create a dynamic feature module, go to File > New > New Module in Android Studio. Then select "Dynamic Feature Module" and follow the prompts to create your module. This will create a separate module that contains the code and resources for the dynamic feature.
Configure your app to use dynamic features: In your app-level build.gradle file, add the following code to enable dynamic feature delivery:
android {
...
dynamicFeatures = [":dynamicfeature"]
}
dependencies {
...
implementation "com.google.android.play:core:1.8.1"
}
Replace :dynamicfeature with the name of your dynamic feature module. This code tells the Google Play Core library to handle dynamic feature delivery for your app.
Implement feature modules in your app code: In your app code, you can check if a specific dynamic feature is installed and available on the user's device using the SplitInstallManager API. Here's an example:
val splitInstallManager = SplitInstallManagerFactory.create(context)
val request = SplitInstallRequest.newBuilder()
.addModule("dynamicfeature")
.build()
splitInstallManager.startInstall(request)
.addOnSuccessListener { result ->
// Feature module installed successfully
}
.addOnFailureListener { exception ->
// Feature module installation failed
}
This code checks if the dynamicfeature module is installed on the user's device and, if not, requests that it be downloaded and installed. Once the installation is complete, the app can use the code and resources in the dynamic feature module.
By using dynamic features in your Android app, you can significantly reduce the size of your app and improve its installation time, which can lead to a better user experience. However, it's important to carefully consider which parts of your app should be delivered as dynamic features to ensure that they are used frequently enough to justify the added complexity.
Conclusion
Reducing the size of your Android app can significantly improve user experience and increase user retention. Use the tips and tricks discussed in this post to optimize your app's size while maintaining functionality and performance. Remember to test your app thoroughly after making any changes to ensure that it works as expected.
Comments