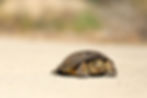
Flutter, Google's open-source UI development framework, has gained immense popularity among developers for its cross-platform capabilities and smooth performance. However, like any software development framework, Flutter apps may encounter frame rate issues that can impact user experience.
In this blog, we will explore the common causes of frame rate issues in Flutter apps and provide effective solutions to mitigate them.
Understanding Frame Rate Issues in Flutter Apps
The frame rate of a Flutter app refers to the number of frames or screen updates displayed per second. The standard frame rate for smooth user experience is 60 frames per second (fps). If an app fails to achieve this frame rate consistently, it can result in stuttering animations, sluggish responsiveness, and an overall degraded user experience.
In Android, frame rate issues may manifest as App Not Responding (ANR) if the UI Thread gets blocked for 5000 milliseconds or more. If the UI Frames take 700 milliseconds or more to render it is a Frozen Frame situation and if it takes 16 milliseconds or more it is a Slow Frame situation.
In iOS, if the UI Thread is stuck for 250 milliseconds or more it is an App Hang, also called App Freeze, situation.
Common Causes of Frame Rate Issues
1. Expensive Widget Rebuilds
class MyExpensiveWidget extends StatelessWidget {
final ExpensiveData data;
const MyExpensiveWidget({required this.data});
@override
Widget build(BuildContext context) {
// Widget build logic that might be expensive
return ...;
}
}
To optimize widget rebuilds, use const constructors whenever possible. By using const, Flutter can efficiently skip the widget rebuild if the constructor parameters haven't changed.
2. Inefficient Animations
class MyAnimationWidget extends StatefulWidget {
@override
_MyAnimationWidgetState createState() => _MyAnimationWidgetState();
}
class _MyAnimationWidgetState extends State<MyAnimationWidget>
with SingleTickerProviderStateMixin {
late AnimationController _controller;
late Animation<double> _animation;
@override
void initState() {
super.initState();
_controller = AnimationController(
duration: const Duration(milliseconds: 500),
vsync: this,
);
_animation = Tween(begin: 0.0, end: 1.0).animate(_controller);
_controller.forward();
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return AnimatedBuilder(
animation: _animation,
builder: (context, child) {
// Widget build logic using the animation value
return ...;
},
);
}
}
To optimize animations, use lightweight animations like Tween animations instead of heavy ones like Hero animations. Properly dispose of animation controllers to release resources and avoid unnecessary computations. Implement animation caching techniques, such as pre-loading and reusing animations, to reduce performance impact.
3. Inadequate Caching and Data Fetching
class MyDataFetcher {
static final Map<String, dynamic> _cache = {};
static Future<dynamic> fetchData(String url) async {
if (_cache.containsKey(url)) {
return _cache[url];
} else {
final response = await http.get(Uri.parse(url));
final data = json.decode(response.body);
_cache[url] = data;
return data;
}
}
}
To optimize caching and data fetching, implement proper caching strategies. Utilize Flutter's built-in caching mechanisms, such as cached_network_image, to minimize repeated image downloads. Implement pagination techniques to fetch data incrementally instead of in one large chunk.
4. Simplify Layouts
class MyComplexLayout extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Container(
child: Column(
children: [
Expanded(
child: Row(
children: [
Flexible(child: Container()),
Flexible(child: Container()),
],
),
),
Expanded(
child: Container(),
),
],
),
);
}
}
To simplify layouts, minimize nested layouts and unnecessary constraints. Use appropriate layout widgets based on specific requirements. Avoid excessive use of Expanded and Flexible widgets when other layout techniques like SizedBox or AspectRatio can achieve the desired results.
Use App Performance Monitoring (APM) Tools
Monitoring the frame rate of a Flutter app is crucial for maintaining optimal performance and delivering a smooth user experience. APM tools provide valuable insights into the app's rendering performance, allowing developers to identify and address frame rate issues effectively.
Two widely used tools for frame rate monitoring in Flutter are Firebase Performance Monitoring and Finotes.
Conclusion
Frame rate issues in Flutter apps can negatively impact the user experience, leading to reduced engagement and user satisfaction. By optimizing widget rebuilds, animations, caching and data fetching, as well as simplifying layouts, developers can ensure a smooth and responsive UI.
Remember to profile your app, optimize animations, simplify layouts, and follow best practices to address frame rate issues effectively. Use APM tools to continuously monitor app performance including frame rate issues. With careful attention to performance optimization, Flutter can deliver exceptional user experiences across various platforms.