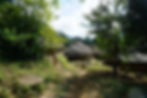
In today's mobile app development landscape, building data-driven applications is a common requirement. To efficiently handle data fetching and manipulation, it's crucial to have a robust API layer that simplifies the communication between the frontend and backend.
GraphQL, a query language for APIs, and Hasura, an open-source GraphQL engine, offer a powerful combination for building data-driven Flutter apps. In this blog post, we will explore how to integrate GraphQL with Flutter using Hasura and leverage its features to create efficient and scalable apps.
Prerequisites
To follow along with this tutorial, you should have the following prerequisites:
Basic knowledge of Flutter and Dart.
Flutter SDK installed on your machine.
An existing Flutter project or create a new one using flutter create my_flutter_app.
Set up Hasura GraphQL Engine
Before integrating GraphQL with Flutter, we need to set up the Hasura GraphQL Engine to expose our data through a GraphQL API. Here's a high-level overview of the setup process:
1. Install Hasura GraphQL Engine:
Option 1: Using Docker:
Install Docker on your machine if you haven't already.
Pull the Hasura GraphQL Engine Docker image using the command: docker pull hasura/graphql-engine.
Start the Hasura GraphQL Engine container: docker run -d -p 8080:8080 hasura/graphql-engine.
Option 2: Using Hasura Cloud:
Visit the Hasura Cloud website (https://hasura.io/cloud) and sign up for an account.
Create a new project and follow the setup instructions provided.
2. Set up Hasura Console
Access the Hasura Console by visiting http://localhost:8080 or your Hasura Cloud project URL.
Authenticate with the provided credentials (default is admin:admin).
Create a new table or use an existing one to define your data schema.
3. Define GraphQL Schema
Use the Hasura Console to define your GraphQL schema by auto-generating it from an existing database schema or manually defining it using the GraphQL SDL (Schema Definition Language).
4. Explore GraphQL API
Once the schema is defined, you can explore the GraphQL API by executing queries, mutations, and subscriptions in the Hasura Console.
Congratulations! You have successfully set up the Hasura GraphQL Engine. Now, let's integrate it into our Flutter app.
Add Dependencies
To use GraphQL in Flutter, we need to add the necessary dependencies to our pubspec.yaml file. Open the file and add the following lines:
dependencies:flutter:sdk: fluttergraphql_flutter: ^5.1.2
Save the file and run flutter pub get to fetch the dependencies.
Create GraphQL Client
To interact with the Hasura GraphQL API, we need to create a GraphQL client in our Flutter app. Create a new file, graphql_client.dart, and add the following code:
import 'package:graphql_flutter/graphql_flutter.dart';
class GraphQLService {
static final HttpLink httpLink = HttpLink('http://localhost:8080/v1/graphql');
static final GraphQLClient client = GraphQLClient(
link: httpLink,
cache: GraphQLCache(),
);
}
In the above code, we define an HTTP link to connect to our Hasura GraphQL API endpoint. You may need to update the URL if you are using Hasura Cloud or a different port. We then create a GraphQL client using the GraphQLClient class from the graphql_flutter package.
Query Data from Hasura
Now, let's fetch data from the Hasura GraphQL API using our GraphQL client. Update your main Flutter widget (main.dart) with the following code:
import 'package:flutter/material.dart';
import 'package:graphql_flutter/graphql_flutter.dart';
import 'graphql_client.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return GraphQLProvider(
client: GraphQLService.client,
child: MaterialApp(
title: 'Flutter GraphQL Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(),
),
);
}
}
class MyHomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('GraphQL Demo'),
),
body: Query(
options: QueryOptions(
document: gql('YOUR_GRAPHQL_QUERY_HERE'),
),
builder: (QueryResult result, {VoidCallback? refetch}) {
if (result.hasException) {
return Text(result.exception.toString());
}
if (result.isLoading) {
return CircularProgressIndicator();
}
// Process the result.data object and display the data in your UI
// ...
return Container();
},
),
);
}
}
In the above code, we wrap our Flutter app with the GraphQLProvider widget, which provides the GraphQL client to all descendant widgets. Inside the MyHomePage widget, we use the Query widget from graphql_flutter to execute a GraphQL query. Replace 'YOUR_GRAPHQL_QUERY_HERE' with the actual GraphQL query you want to execute.
Display Data in the UI
Inside the builder method of the Query widget, we can access the query result using the result parameter. Process the result.data object to extract the required data and display it in your UI. You can use any Flutter widget to display the data, such as Text, ListView, or custom widgets.
Congratulations! You have successfully integrated GraphQL with Flutter using Hasura. You can now fetch and display data from your Hasura GraphQL API in your Flutter app.
Conclusion
In this blog post, we explored how to integrate GraphQL with Flutter using Hasura. We set up the Hasura GraphQL Engine, created a GraphQL client in Flutter, queried data from the Hasura GraphQL API, and displayed it in the UI.
By leveraging the power of GraphQL and the simplicity of Hasura, you can build efficient and scalable data-driven apps with Flutter.
Remember to handle error scenarios, mutations, and subscriptions based on your app requirements. Explore the graphql_flutter package documentation for more advanced usage and features.
Happy coding!