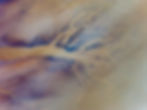
GraphQL is a powerful query language for APIs that provides a flexible and efficient way to fetch data. In this tutorial, we will explore how to integrate and use GraphQL in Android apps using the Hasura, Apollo library and Kotlin.
In this blog we'll learn how to create a GraphQL schema, implement a GraphQL client, and perform CRUD operations on todo items.
Prerequisites
To follow this tutorial, you will need the following prerequisites:
An Android Studio IDE: Install Android Studio from the official website (https://developer.android.com/studio) and set it up on your system.
A basic understanding of Kotlin: Familiarize yourself with the Kotlin programming language, as this tutorial assumes basic knowledge of Kotlin syntax and concepts.
An Apollo account: Sign up for an account on the Apollo platform (https://www.apollographql.com/) to set up and manage your GraphQL API.
A Hasura account: Create an account on Hasura (https://hasura.io/) to set up your Hasura GraphQL server.
Creating a New Project
Open Android Studio and create a new Android project with an appropriate name and package. Configure the project settings, such as the minimum SDK version and activity template, according to your preferences.
Adding Dependencies
Open the project's build.gradle file. In the dependencies block, add the following dependencies:
dependencies {
implementation 'com.apollographql.apollo:apollo-runtime:1.0.1-SNAPSHOT'
compileOnly 'org.jetbrains:annotations:13.0'
testCompileOnly 'org.jetbrains:annotations:13.0'
}
Sync the project to download the required dependencies.
Creating a GraphQL Schema
Create a new file in your project's directory called api.graphql. In this file, define the GraphQL schema that describes the structure of the data you'll be fetching from the Hasura server.
Here's the schema for a Todo app:
schema {
query: Query
mutation: Mutation
}
type Query {
allTodos: [Todo]
searchTodos(text: String!): [Todo]
}
type Mutation {
createTodo(text: String!): Todo
updateTodo(id: ID!, text: String!): Todo
deleteTodo(id: ID!): Todo
}
type Todo {id: ID!text: String
completed: Boolean
}
Please note that the text argument is marked with an exclamation mark (!), indicating that it is a required field.
Creating a GraphQL Client
Create a new Kotlin file in your project's directory called GraphQLClient.kt. Inside the GraphQLClient class, define functions that will handle making requests to the Hasura server and fetching data.
Here's an example implementation:
import com.apollographql.apollo.ApolloClient
class GraphQLClient {
private val apolloClient = ApolloClient.Builder()
.serverUrl("https://api.hasura.io/v1/graphql")
.build()
fun allTodos(): List<Todo> {
val query = """
query allTodos {
todos {
id
text
completed
}
}
"""
val result = apolloClient.query(query).execute()
return result.data?.todos ?: emptyList()
}
fun createTodo(text: String): Todo {
val mutation = """
mutation createTodo($text: String!) {
createTodo(text: $text) {
id
text
completed
}
}
"""
val result = apolloClient.mutate(mutation).execute()
return result.data?.createTodo ?: Todo()
}
fun searchTodos(text: String): List<Todo> {
val query = """
query searchTodos($text: String!) {
todos(where: { text: { contains: $text } }) {
id
text
completed
}
}
"""
val result = apolloClient.query(query).execute()
return result.data?.todos ?: emptyList()
}
fun updateTodo(id: String, text: String): Todo {
val mutation = """
mutation updateTodo($id: ID!, $text: String!) {
updateTodo(id: $id, text: $text) {
id
text
completed
}
}
"""
val result = apolloClient.mutate(mutation).execute()
return result.data?.updateTodo ?: Todo()
}
fun deleteTodo(id: String): Todo {
val mutation = """
mutation deleteTodo($id: ID!) {
deleteTodo(id: $id) {
id
text
completed
}
}
"""
val result = apolloClient.mutate(mutation).execute()
return result.data?.deleteTodo ?: Todo()
}
}
Using the GraphQL Client
Now that we have a GraphQL client, we can use it to fetch data from the Hasura server and perform CRUD operations on todo items. In your activity or fragment code, create an instance of the GraphQLClient class and call the desired functions to interact with the data.
Here's an example:
val graphQLClient = GraphQLClient()
// Fetch all todo items
val todos = graphQLClient.allTodos()
// Create a new todo item
val createdTodo = graphQLClient.createTodo("Buy groceries")
// Search for todo items containing a specific text
val searchedTodos = graphQLClient.searchTodos("groceries")
// Update a todo item
val updatedTodo = graphQLClient.updateTodo(createdTodo.id, "Buy milk and eggs")
// Delete a todo item
val deletedTodo = graphQLClient.deleteTodo(updatedTodo.id)
Customize the code as per your application's requirements, such as displaying the fetched data in a RecyclerView or handling errors and edge cases.
Conclusion
In this blog, we learned how to integrate and use GraphQL in Android apps using Apollo and Kotlin. We started by creating a new Android Studio project and adding the necessary dependencies. Then, we created a GraphQL schema and implemented a GraphQL client using the Apollo library. Finally, we used the GraphQL client to fetch data from the Hasura server and perform CRUD operations on todo items.
GraphQL offers a powerful and flexible approach to fetching data, allowing you to retrieve only the data you need in a single request. By leveraging the Apollo library and Kotlin, you can easily integrate GraphQL into your Android apps and build efficient data-fetching solutions.
I hope you found this blog helpful. If you have any further questions, please feel free to leave a comment below.