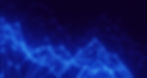
Firebase Firestore is a cloud-based NoSQL database that allows you to store and retrieve data in real time. It is an excellent choice for iOS apps due to its ease of use, scalability, and security.
In this blog post, we will guide you through the process of integrating Firestore with Swift and demonstrate how to leverage its features in iOS development.
Adding Firebase to Your iOS Project
To begin, you need to add Firebase to your iOS project. Follow the instructions provided in the Firebase documentation (https://firebase.google.com/docs/ios/setup) to complete this step.
Once you have successfully added Firebase to your project, you must import the FirebaseFirestoreSwift framework. To do this, add the following line to your Podfile:
pod 'FirebaseFirestoreSwift'
Mapping Firestore Data to Swift Types
Firestore data is stored in documents, which are essentially JSON objects. You can map Firestore documents to Swift types by utilizing the Codable protocol.
To map a Firestore document to a Swift type, your type declaration should conform to Codable. Add the following two lines to your type declaration:
import Codable
@objc(MyDocument)struct MyDocument: Codable {
// ...
}
By adopting the Codable protocol, you gain access to a range of methods for encoding and decoding JSON objects. These methods will facilitate the reading and writing of data to Firestore.
Reading and Writing Data to Firestore
After successfully mapping your Firestore data to Swift types, you can commence reading and writing data to Firestore.
To read data from Firestore, utilize the DocumentReference class. This class offers several methods for obtaining, setting, and deleting data from Firestore documents.
For instance, the following code retrieves data from a Firestore document:
let document = Firestore.firestore().document("my-document")
let data = try document.data(as: MyDocument.self)
To write data to Firestore, make use of the setData() method on the DocumentReference class. This method accepts a dictionary of key-value pairs as its argument.
For example, the following code writes data to a Firestore document:
let document = Firestore.firestore().document("my-document")
document.setData(["name": "Robin", "age": 30])
Using Firestore in a Real-Time App
Firestore is a real-time database, meaning that any changes made to the data are instantly reflected across all connected clients. This real-time capability makes Firestore an ideal choice for developing real-time apps.
To incorporate Firestore into a real-time app, employ the Listener class. This class provides a mechanism for listening to changes in Firestore data.
For instance, the following code sets up a listener to monitor changes in a Firestore document:
let document = Firestore.firestore().document("my-document")
let listener = document.addSnapshotListener { snapshot, error inif let error = error {
// Handle the error
} else {
// Update the UI with new data
}
}
Conclusion
In this blog post, we explored the process of integrating Firestore with Swift and demonstrated its utilization in iOS development.
We hope this blog post has provided you with a solid foundation for working with Firestore in Swift.
Happy Coding!