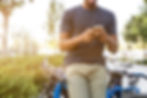
Push notifications are a crucial component of modern mobile applications, allowing you to engage and re-engage users by sending timely updates and reminders.
In this blog post, we'll explore how to integrate push notifications in a Flutter app using Firebase Cloud Messaging (FCM). Firebase Cloud Messaging is a powerful and user-friendly platform that enables sending notifications to both Android and iOS devices.
Prerequisites
Before we begin, ensure that you have the following prerequisites in place:
Flutter Development Environment: Make sure you have Flutter and Dart installed on your system. If not, follow the official Flutter installation guide: Flutter Installation Guide
Firebase Project: Create a Firebase project if you haven't already. Visit the Firebase Console (https://console.firebase.google.com/) and set up a new project.
Step 1: Set Up Firebase Project
Go to the Firebase Console and select your project.
Click on "Project settings" and then navigate to the "Cloud Messaging" tab.
Here, you'll find your Server Key and Sender ID. These will be used later in your Flutter app to communicate with Firebase Cloud Messaging.
Step 2: Add Firebase Dependencies
In your Flutter project, open the pubspec.yaml file and add the necessary Firebase dependencies:
dependencies:
flutter:
sdk: flutter
firebase_core: ^1.12.0
firebase_messaging: ^11.1.0
After adding the dependencies, run flutter pub get to fetch them.
Step 3: Initialize Firebase
Open your main.dart file and initialize Firebase in the main function:
import 'package:flutter/material.dart';
import 'package:firebase_core/firebase_core.dart';
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
runApp(MyApp());
}
Step 4: Request Notification Permissions
To receive push notifications, you need to request user permission. Add the following code to your main widget (usually MyApp):
import 'package:firebase_messaging/firebase_messaging.dart';
class MyApp extends StatelessWidget {
final FirebaseMessaging _firebaseMessaging = FirebaseMessaging.instance;
@override
Widget build(BuildContext context) {
// Request notification permissions
_firebaseMessaging.requestPermission();
return MaterialApp(
// ...
);
}
}
Step 5: Handle Notifications
Now let's handle incoming notifications. Add the following code to the same widget where you requested permissions:
class MyApp extends StatelessWidget {
final FirebaseMessaging _firebaseMessaging = FirebaseMessaging.instance;
@override
void initState() {
super.initState();
// Handle incoming messages
FirebaseMessaging.onMessage.listen((RemoteMessage message) {
// Handle the message
print("Received message: ${message.notification?.title}");
});
}
@override
Widget build(BuildContext context) {
// ...
}
}
Step 6: Displaying Notifications
To display notifications when the app is in the background or terminated, you need to set up a background message handler. Add the following code to your main widget:
class MyApp extends StatelessWidget {
final FirebaseMessaging _firebaseMessaging = FirebaseMessaging.instance;
@override
void initState() {
super.initState();
FirebaseMessaging.onMessage.listen((RemoteMessage message) {
print("Received message: ${message.notification?.title}");
});
// Handle messages when the app is in the background or terminated
FirebaseMessaging.onBackgroundMessage(_firebaseMessagingBackgroundHandler);
}
// Define the background message handler
Future<void> _firebaseMessagingBackgroundHandler(RemoteMessage message) async {
print("Handling a background message: ${message.notification?.title}");
}
@override
Widget build(BuildContext context) {
// ...
}
}
Step 7: Sending Test Notifications
Now that your Flutter app is set up to receive notifications, let's test it by sending a test notification from the Firebase Console:
Go to the Firebase Console and select your project.
Navigate to the "Cloud Messaging" tab.
Click on the "New Notification" button.
Enter the notification details and target your app.
Click "Send Test Message."
Conclusion
Congratulations! You've successfully integrated push notifications in your Flutter app using Firebase Cloud Messaging. You've learned how to request notification permissions, handle incoming messages, and set up background message handling. This capability opens up a world of possibilities for engaging your users and providing timely updates.
Firebase Cloud Messaging provides even more features, such as sending notifications to specific topics, customizing notification appearance, and handling user interactions with notifications. Explore the Firebase Cloud Messaging documentation to learn more about these advanced features and take your app's notification experience to the next level.
Happy coding!