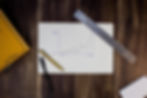
Charts are indispensable tools for visualizing data and conveying complex information in a clear and concise manner. With SwiftUI, you have the power to create stunning user interfaces, and thanks to the Charts library, adding interactive and visually appealing charts to your iOS apps has never been easier.
In this blog post, we will explore how to seamlessly integrate charts into SwiftUI applications and demonstrate the creation of various chart types. We'll also delve into customizing the appearance of your charts to make them stand out. So, let's get started!
Importing the Charts Library
Before we dive into chart creation, the first step is to import the Charts library into your SwiftUI project. You can do this by simply adding the following import statement to your Swift file:
import Charts
With this done, you're ready to start building your charts.
Creating a Simple Bar Chart
Let's begin with a straightforward example of a bar chart. Imagine you want to display the number of sales for each month of the year. Here's how you can create a bar chart using SwiftUI and the Charts library:
struct BarChartView: View {
var body: some View {
Chart {
// Add the bar marks
BarMarks(data: [
(1, 10),
(2, 20),
(3, 30),
(4, 40),
(5, 50),
(6, 60),
])
}
}
}
In this code, we define a BarChartView that embeds a Chart containing BarMarks. The BarMarks mark takes an array of data points, where each point is a tuple consisting of an x-axis value (in this case, the months of the year) and a y-axis value (the number of sales for each month).
Creating Other Types of Charts
The Charts library supports a variety of chart types, including line charts, pie charts, and scatter plots. Let's take a look at how to create each of these:
Line Chart
LineChart(data: [
(1, 10),
(2, 20),
(3, 30),
(4, 40),
(5, 50),
(6, 60),
])
Pie Chart
PieChart(data: [
(10, "Red"),
(20, "Green"),
(30, "Blue"),
])
Scatter Plot
ScatterPlot(data: [
(1, 10),
(2, 20),
(3, 30),
(4, 40),
(5, 50),
(6, 60),
])
You can choose the chart type that best suits your data visualization needs.
Customizing the Appearance of Charts in SwiftUI
To make your charts more visually appealing and aligned with your app's design, you can customize various aspects of their appearance. Here's an example of how to customize a bar chart:
BarChart(data: [
(1, 10),
(2, 20),
(3, 30),
(4, 40),
(5, 50),
(6, 60),
]) {
// Change the bar colors
barFill = Color.red
barStroke = Color.black
// Change the font size of the axis labels
axisLabels.font = .system(size: 12)
}
In this snippet, we modify the bar colors to red, set the bar stroke color to black, and adjust the font size of the axis labels to 12 points. These customizations help you tailor the charts to your specific design requirements.
Conclusion
In this comprehensive guide, we've covered the process of integrating charts into your SwiftUI apps using the Charts library. You've learned how to create various chart types, including bar charts, line charts, pie charts, and scatter plots, as well as how to customize their appearance to make them visually appealing. With these skills, you can enhance your app's user experience and effectively convey data-driven insights to your users.
We hope this tutorial has equipped you with the knowledge and tools to leverage charts in your SwiftUI applications. Feel free to explore additional resources and documentation to further enhance your charting capabilities and create stunning data visualizations in your apps.
Happy coding!