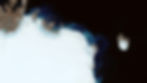
Picture this: You are developing a slick new Kotlin-based Android app. The UI is coming together nicely, but those image thumbnails just won't agree to load as quickly as you would like them to. How to solve this?
Enter Coil, a high-performance library for image loading.
We will jump the gun here to tell you how to go about integrating Coil into your Kotlin-based Apps.
Integrating Coil in Android Kotlin Apps
Integrating Coil onto your Android project using Maven-based dependency management is as easy as 1-2-3. Coil is available by calling the function mavenCentral(). Add the Coil Dependency to your build.gradle file. Open your module-level build.gradle and add the Coil dependency.
dependencies {
implementation(“io.coil-kt:coil:x.y.z”) // Use the latest version
}
Jetpack Compose on Coil
Using Jetpack Compose for building your UI and integrating with Coil for image loading comes with its advantages. This modern Android UI toolkit is designed to simplify and accelerate UI development on Android. Simply, Import the Jetpack Compose extension library and use the following code:
implementation("io.coil-kt:coil-compose:x.y.z")
And later to use the AsyncImage composable which comes as a part of coil-compose, to load an image, use the:
AsyncImage(
model = "https://example.com/image.jpg",
contentDescription = null
)
Why use Coil in Android Apps?
Now that we have spoken in detail and how easily you can integrate Coil, let’s also understand why you as an Android App Developer should use Coil. We will fast forward to the functionalities of Coil and how features like memory and disk caching alongside customisations help achieve minimal boilerplate.
Fast Image Loading: Coil helps avoid the complexities of handling image loading manually by focusing on efficiently loading and caching images from various sources, such as URLs, local files etc. This simplistic feature avoids verbose code or any complex configurations.
// Example of loading an image with Coil
val imageView: ImageView = findViewById(R.id.imageView)
val imageUrl = "https://example.com/image.jpg"
imageView.load(imageUrl)
Built-in Transformation Support: Coil allows developers to apply various modifications to images, such as resizing, cropping, or applying filters. This reduces the need for additional code when manipulating images.
// Example with image transformations
imageView.load(imageUrl) {
transformations(CircleCropTransformation())
}
Disk Caching: Caching reduces the need to repeatedly download or load images, enhancing the overall responsiveness of the app.
Automatic Request Management: Coil handles the retrieval, decoding, and displaying of the image without requiring extensive manual intervention.
ImageView Integration: With Coil, you can easily load an image into an ImageView using Coil's API, making it straightforward to display images in UI components.
// Example of loading an image with Coil
val imageView: ImageView = findViewById(R.id.imageView)
val imageUrl = "https://example.com/image.jpg"
imageView.load(imageUrl)
Customisation & Configuration: Developers can configure options such as placeholder images, error images, and image transformations to meet specific requirements.
// Example with placeholder and error handling
imageView.load(imageUrl) {
placeholder(R.drawable.placeholder)
error(R.drawable.error)
}
Small Library Size: Coil is designed to be lightweight, making it beneficial for projects where minimising the app's size is a priority. It also makes use of modern libraries including Coroutines, OkHttp, Okio, and AndroidX Lifecycles.
Kotlin-Friendly API: Coil is written in Kotlin and offers a Kotlin-friendly API, making it particularly well-suited for projects developed in Kotlin.
For more info and use cases that would make your life easier as a developer, do check out this link. This has an entire repository on how to seamlessly integrate Coil into your Apps. Happy Coding!