Integrating Google Maps in Jetpack Compose Android Apps
- Sep 6, 2023
- 3 min read
Updated: Sep 7, 2023
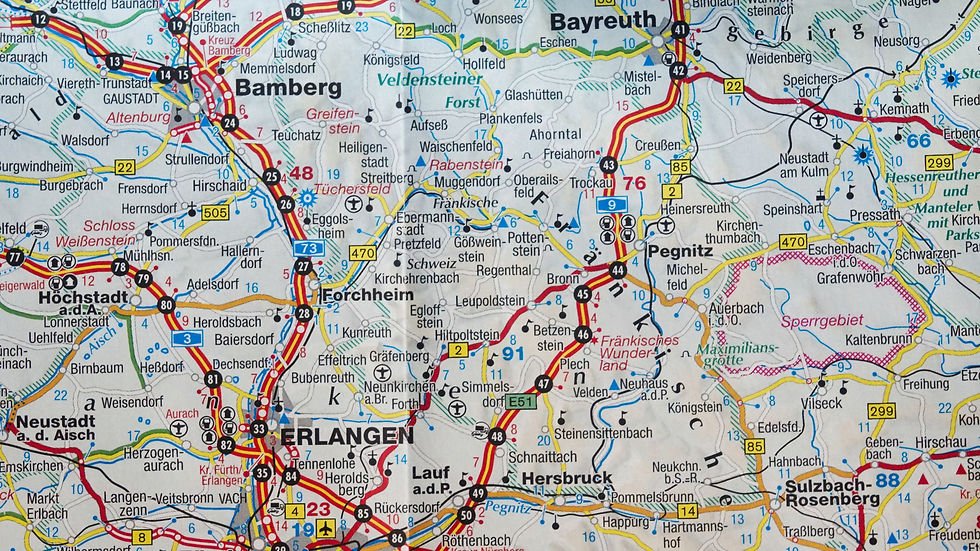
Are you looking to add Google Maps integration to your Jetpack Compose Android app and display a moving vehicle on the map?
You're in the right place!
In this step-by-step guide, we'll walk you through the process of setting up Google Maps in your Android app using Jetpack Compose and adding a dynamic moving vehicle marker.
Prerequisites
Before we dive into the implementation, make sure you have the following prerequisites in place:
Android Studio Arctic Fox: Ensure you have the latest version of Android Studio installed.
Google Maps Project: Create a Google Maps project in Android Studio using the "Empty Compose Activity" template. This template automatically includes the necessary dependencies for Jetpack Compose.
Google Maps API Key: You'll need a Google Maps API key for your project.
Now, let's get started with the integration:
Step 1: Set Up the Android Project
Open Android Studio and create a new Jetpack Compose project.
In the build.gradle (Project) file, add the Google Maven repository:
allprojects {
repositories {
// other repositories
google()
}
}
In the build.gradle (app) file, add the dependencies for Jetpack Compose, Google Maps, and Permissions:
android {
// ...
defaultConfig {
// ...
// Add the following line
resValue "string", "google_maps_api_key", "{YOUR_API_KEY}"
}
// ...
}
dependencies {
// ...
// Google Maps
implementation "com.google.android.gms:play-services-maps:18.1.0"
implementation "com.google.maps.android:maps-ktx:3.2.1"
// Permissions
implementation "com.permissionx.guolindev:permissionx:1.7.0"
}
Replace {YOUR_API_KEY} with your actual Google Maps API key.
Step 2: Request Location Permissions
In your Compose activity or fragment, request location permissions from the user using PermissionX or any other permission library of your choice.
import com.permissionx.guolindev.PermissionX
// Inside your Composable function
PermissionX.init(this@YourActivity)
.permissions(Manifest.permission.ACCESS_FINE_LOCATION)
.request { granted, _, _ ->
if (granted) {
// User granted location permission
} else {
// Handle permission denied
}
}
Step 3: Create a Map Composable
Now, let's create a Composable function to display the Google Map.
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.viewinterop.AndroidView
import com.google.android.gms.maps.CameraUpdateFactory
import com.google.android.gms.maps.GoogleMap
import com.google.android.gms.maps.MapView
import com.google.android.gms.maps.model.LatLng
import com.google.android.gms.maps.model.MarkerOptions
@Composable
fun MapView() {
val mapView = rememberMapViewWithLifecycle()
AndroidView(
modifier = Modifier.fillMaxSize(),
factory = { context ->
mapView.apply {
// Initialize the MapView
onCreate(null)
getMapAsync { googleMap ->
// Set up Google Map settings here
val initialLocation = LatLng(37.7749, -122.4194) // Default location (San Francisco)
googleMap.moveCamera(CameraUpdateFactory.newLatLngZoom(initialLocation, 12f))
// Add a marker for the vehicle
val vehicleLocation = LatLng(37.7749, -122.4194) // Example vehicle location
val vehicleMarker = MarkerOptions().position(vehicleLocation).title("Vehicle")
googleMap.addMarker(vehicleMarker)
}
}
}
)
}
Replace the default and example coordinates with the desired starting location for your map and the initial vehicle position.
Step 4: Animate the Vehicle
To animate the vehicle, you'll need to update its position periodically. You can use Handler or a timer for this purpose. Here's a simplified example of how to animate the vehicle:
import android.os.Handler
import androidx.compose.runtime.*
@Composable
fun MapWithAnimatedVehicle() {
val mapView = rememberMapViewWithLifecycle()
var vehicleLocation by remember { mutableStateOf(LatLng(37.7749, -122.4194)) }
AndroidView(
modifier = Modifier.fillMaxSize(),
factory = { context ->
mapView.apply {
// Initialize the MapView
onCreate(null)
getMapAsync { googleMap ->
// Set up Google Map settings here
googleMap.moveCamera(CameraUpdateFactory.newLatLngZoom(vehicleLocation, 12f))
// Add a marker for the vehicle
val vehicleMarker = MarkerOptions().position(vehicleLocation).title("Vehicle")
googleMap.addMarker(vehicleMarker)
// Animate the vehicle's movement
val handler = Handler()
val runnable = object : Runnable {
override fun run() {
// Update the vehicle's position (e.g., simulate movement)
vehicleLocation = LatLng(
vehicleLocation.latitude + 0.001,
vehicleLocation.longitude + 0.001
)
googleMap.animateCamera(
CameraUpdateFactory.newLatLng(vehicleLocation)
)
handler.postDelayed(this, 1000) // Update every 1 second
}
}
handler.post(runnable)
}
}
}
)
}
This code sets up a simple animation that moves the vehicle marker by a small amount every second. You can customize this animation to fit your specific use case.
Step 5: Display the Map in Your UI
Finally, you can use the MapView or MapWithAnimatedVehicle Composable functions within your Compose UI hierarchy to display the map. For example:
@Composable
fun YourMapScreen() {
Column {
// Other Composables and UI elements
MapWithAnimatedVehicle()
// Other Composables and UI elements
}
}
That's it! You've successfully integrated Google Maps into your Jetpack Compose Android app and animated a moving vehicle marker on the map.
Conclusion
In this blog post, we've covered the basics of integrating Google Maps into your Jetpack Compose Android app and added a dynamic moving marker. You can further enhance this example by integrating location tracking, route rendering, and more, depending on your project requirements.
I hope this guide was helpful in getting you started with Google Maps in Jetpack Compose. If you have any questions or need further assistance, please don't hesitate to ask.
Happy coding!
Comments