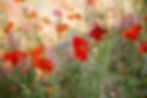
In Flutter 3.10, an exciting change was introduced to the way MediaQuery is handled. MediaQuery, which provides access to the media information of the current context, was transformed into an InheritedModel. This change simplifies the process of accessing MediaQueryData throughout your Flutter application.
In this blog post, we will explore the implications of this change and how it affects the way we work with MediaQuery in Flutter.
Understanding InheritedModel
Before diving into the specifics of how MediaQuery became an InheritedModel, let's briefly understand what InheritedModel is in Flutter. InheritedModel is a Flutter widget that allows the propagation of data down the widget tree. It provides a way to share data with descendant widgets without having to pass it explicitly through constructors.
In previous versions of Flutter, MediaQuery was not an InheritedModel, meaning that accessing MediaQueryData in nested widgets required some extra steps. However, starting from Flutter 3.10, MediaQuery became an InheritedModel, streamlining the process of accessing and using media-related information across your app.
Simplified Access to MediaQueryData
With the migration of MediaQuery to an InheritedModel, accessing MediaQueryData became much simpler. Previously, you needed to use a StatefulWidget and a GlobalKey to store and retrieve MediaQueryData. However, after Flutter 3.10, you can directly use the MediaQuery.of(context) method to access the MediaQueryData for the current context.
The new approach allows you to obtain MediaQueryData anywhere in your widget tree without the need for additional boilerplate code. Simply provide the appropriate context, and you will have access to valuable information such as the size, orientation, and device pixel ratio.
Benefits of InheritedModel
The shift of MediaQuery to an InheritedModel offers several benefits for Flutter developers:
Simplified Code: The direct usage of MediaQuery.of(context) eliminates the need for GlobalKey and StatefulWidget, resulting in cleaner and more concise code.
Improved Performance: As an InheritedModel, MediaQuery optimizes the propagation of changes to MediaQueryData throughout the widget tree. This means that only the necessary widgets will be rebuilt when media-related information changes, resulting in improved performance.
Enhanced Flexibility: By leveraging the InheritedModel approach, you can easily access MediaQueryData from any descendant widget within your app's widget tree. This flexibility enables you to respond dynamically to changes in the device's media attributes and adapt your UI accordingly.
Accessing MediaQueryData Before Flutter 3.10
Before Flutter 3.10, accessing MediaQueryData required the use of a StatefulWidget and GlobalKey.
Let's take a look at the code example:
import 'package:flutter/material.dart';
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
final GlobalKey<_MyAppState> _key = GlobalKey();
MediaQueryData _mediaQueryData;
@override
void initState() {
super.initState();
WidgetsBinding.instance.addPostFrameCallback((_) {
_mediaQueryData = MediaQuery.of(_key.currentContext);
});
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Center(
child: Text(
_mediaQueryData.size.toString(),
),
),
),
);
}
}
In the code snippet above, we define a StatefulWidget, MyApp, which holds a GlobalKey and the MediaQueryData object. Inside the initState method, we access the MediaQuery.of(_key.currentContext) to obtain the MediaQueryData. Finally, in the build method, we display the size of the device screen using the obtained MediaQueryData.
Accessing MediaQueryData in Flutter 3.10
With the introduction of InheritedModel in Flutter 3.10, accessing MediaQueryData became much simpler.
Let's take a look at the updated code example:
import 'package:flutter/material.dart';
void main() {
runApp(
MaterialApp(
home: Scaffold(
body: Center(
child: Builder(
builder: (context) {
final mediaQueryData = MediaQuery.of(context);
return Text(
mediaQueryData.size.toString(),
);
},
),
),
),
),
);
}
In the updated code, we can now directly use MediaQuery.of(context) to access the MediaQueryData within any widget. We use the Builder widget to provide a new BuildContext where we can access the MediaQueryData. Inside the builder function, we obtain the mediaQueryData using MediaQuery.of(context) and display the size of the device screen using a Text widget.
Conclusion
Flutter 3.10 introduced a significant change to the way we access MediaQueryData by transforming MediaQuery into an InheritedModel. This change simplifies the code and eliminates the need for StatefulWidget and GlobalKey to access MediaQueryData. By leveraging the power of InheritedModel, accessing MediaQueryData becomes a straightforward process using MediaQuery.of(context).
As a Flutter developer, staying up-to-date with the latest changes in the framework is crucial. Understanding the migration from StatefulWidget and GlobalKey to InheritedModel ensures that you can write more concise and efficient code. By embracing the simplified approach to accessing MediaQueryData, you can create responsive and adaptable user interfaces in your Flutter applications.