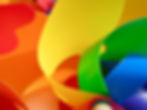
Graphical operations are crucial for creating visually appealing and immersive user experiences in Android app development. However, computationally intensive tasks can strain the device's CPU, leading to slower performance. During early days of Android, developers used Renderscript to implement GPU acceleration and process heavy graphical operations, but it is deprecated now. Now, Developers can leverage the power of the GPU (Graphics Processing Unit) using Vulkan, a low-level graphics API.
In this blog post, we will explore how to utilize GPU capabilities with Vulkan in Kotlin Android apps to efficiently execute heavy graphical operations.
Prerequisites
To follow along with this tutorial, you should have a basic understanding of Android app development using Kotlin. Familiarity with GPU programming concepts and Android Studio will also be helpful.
Step 1: Setting up the Project
Open Android Studio and create a new Android project.
Select the "Empty Activity" template and provide a suitable name for your project.
Choose the minimum API level according to your target audience.
Click "Finish" to create the project.
Step 2: Adding Vulkan Support
Open your app's build.gradle file and add the following line under the android block:
android {
...
defaultConfig {
...
ndk {
// Set the version of the NDK to use
version "your_ndk_version"
}
}
}
Replace "your_ndk_version" with the desired NDK version. Vulkan requires NDK to access low-level GPU capabilities.
Sync your project with Gradle by clicking the "Sync Now" button.
Step 3: Initializing Vulkan
Create a new Kotlin class called VulkanHelper in your project.
Open the VulkanHelper class and define the necessary methods for Vulkan initialization. For example:
import android.content.Context
import android.graphics.Bitmap
import android.util.Log
import org.lwjgl.PointerBuffer
import org.lwjgl.system.MemoryStack
import org.lwjgl.vulkan.*
class VulkanHelper(private val context: Context) {
private lateinit var instance: VkInstance
private lateinit var physicalDevice: VkPhysicalDevice
private lateinit var device: VkDevice
private lateinit var queue: VkQueue
fun initializeVulkan() {
createInstance()
selectPhysicalDevice()
createLogicalDevice()
getDeviceQueue()
}
private fun createInstance() {
val appInfo = VkApplicationInfo.calloc()
.sType(VK11.VK_STRUCTURE_TYPE_APPLICATION_INFO)
.pApplicationName(context.packageName)
.pEngineName("MyEngine")
.apiVersion(VK11.VK_API_VERSION_1_1)
val createInfo = VkInstanceCreateInfo.calloc()
.sType(VK11.VK_STRUCTURE_TYPE_INSTANCE_CREATE_INFO)
.pNext(VK11.VK_NULL_HANDLE)
.pApplicationInfo(appInfo)
val pInstance = MemoryStack.stackPush().use {
val pp = it.mallocPointer(1)
if (VK11.vkCreateInstance(createInfo, null, pp) != VK11.VK_SUCCESS) {
throw RuntimeException("Failed to create Vulkan instance")
}
pp[0]
}
instance = VkInstance(pInstance, createInfo)
appInfo.free()
createInfo.free()
}
private fun selectPhysicalDevice() {
// Select the appropriate physical device based on your requirements// ...
physicalDevice = // Selected physical device
}
private fun createLogicalDevice() {
// Create a logical device using the selected physical device// ...
device = // Created logical device
}
private fun getDeviceQueue() {
val queueFamilyProperties = VkQueueFamilyProperties.malloc(1)
VK11.vkGetPhysicalDeviceQueueFamilyProperties(physicalDevice, queueFamilyProperties)
val pQueue = MemoryStack.stackPush().use {
val pp = it.mallocPointer(1)
VK11.vkGetDeviceQueue(device, 0, 0, pp)
pp[0]
}
queue = VkQueue(pQueue, device)
}
fun performGraphicalOperation(input: Bitmap): Bitmap {
// Perform your heavy graphical operation using Vulkan
// ...
return input
// Placeholder, replace with the processed image
}
fun cleanup() {
// Cleanup Vulkan resources// ...
}
}
Step 4: Integrating Vulkan in your App
Open the desired activity or fragment where you want to use Vulkan for graphical operations.
Inside the activity or fragment, create an instance of the VulkanHelper class.
Call the initializeVulkan() method to initialize Vulkan.
Use the performGraphicalOperation() method to execute heavy graphical operations using Vulkan.
Call the cleanup() method when you're done to release Vulkan resources.
class MainActivity : AppCompatActivity() {
private lateinit var vulkanHelper: VulkanHelper
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
vulkanHelper = VulkanHelper(applicationContext)
vulkanHelper.initializeVulkan()
val inputBitmap: Bitmap = // Obtain or create the input Bitmap
val outputBitmap = vulkanHelper.performGraphicalOperation(inputBitmap)
// Use the outputBitmap for display or further processing
}
override fun onDestroy() {
super.onDestroy()
vulkanHelper.cleanup()
}
}
* Do note that the above code is indicative and is not production ready. You may want to run the operation in a secondary thread and not hog the main thread.
Capabilities of Vulkan
Rendering 3D Graphics: Vulkan provides low-level access to the GPU, allowing developers to efficiently render complex 3D scenes. It supports features like vertex and fragment shaders, texture mapping, lighting effects, and more.
Compute Shaders: Vulkan enables developers to perform highly parallel computations on the GPU using compute shaders. This capability is useful for tasks such as physics simulations, image processing, and artificial intelligence.
Multi-threaded Rendering: Vulkan supports multi-threaded rendering, allowing developers to distribute rendering tasks across multiple CPU cores. This capability improves performance by efficiently utilizing available resources.
Memory Management: Vulkan provides fine-grained control over memory management, allowing developers to allocate, manage, and recycle GPU memory. This capability helps optimize memory usage and improve performance.
Low-Level Control: Vulkan gives developers direct control over GPU operations, reducing overhead and enabling fine-grained optimizations. It provides explicit synchronization mechanisms, memory barriers, and pipeline state management, allowing for efficient command submission and synchronization.
Conclusion
By utilizing Vulkan in Kotlin Android apps, developers can harness the power of GPU for heavy graphical operations. In this tutorial, we explored how to set up the project for Vulkan support, initialize Vulkan using the VulkanHelper class, and integrate Vulkan into an Android activity.
Remember to optimize your Vulkan code for performance and test on different devices to ensure consistent behavior. Leveraging GPU capabilities with Vulkan can significantly enhance the graphical performance of your Android app, resulting in smoother animations and improved user experiences.
Happy coding!